In this blog, I’ve added a list of carefully selected Kubernetes Practice Questions for CKS, CKA, and CKAD certification exams.
This is still a work in progress and I’ll keep adding solutions to the practice questions.
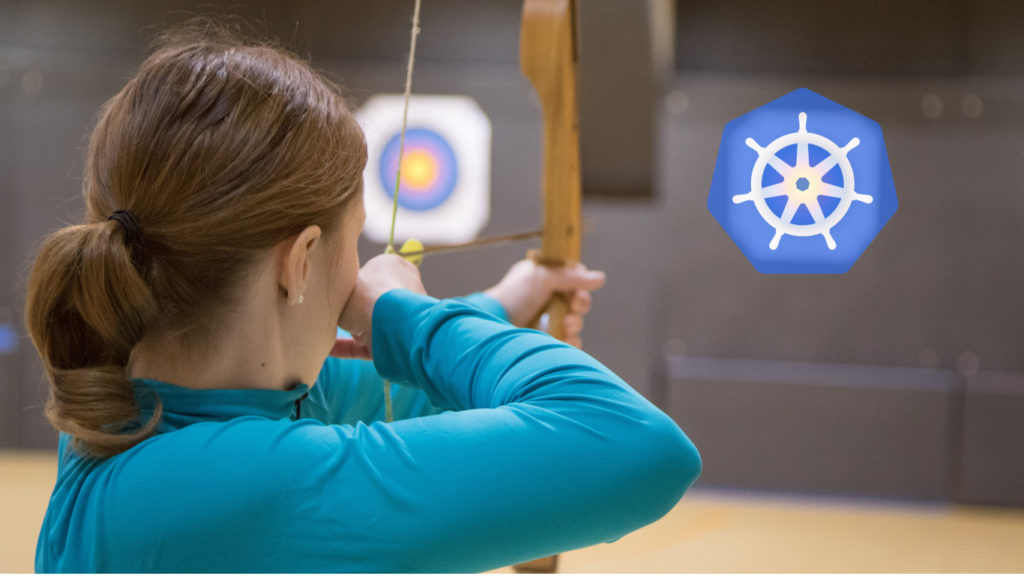
Table of Contents
Practice Makes Kubernetes Skills Perfect
Practice is one of the most essential requirements for learning Kubernetes concepts effectively. It helps you develop practical skills, troubleshooting skills, familiarity with command-line tools, and working with Kubernetes. By engaging in hands-on exercises and regularly practicing Kubernetes concepts, you can become proficient in utilizing its capabilities to effectively manage and orchestrate containerized applications.
Kubernetes Cluster For Practice
You can set up a Single Node Kubernetes Cluster like kind, microk8s, k3s, or a minikube to try most of these questions.
Kubernetes Practice Questions for CKS, CKA, and CKAD
These questions cover all the topics in Kubernetes and are not in any particular order.
Question 1: Write a Kubernetes YAML manifest to create a new namespace named “sandbox” and assign the label “environment=sandbox” to it.
apiVersion: v1
kind: Namespace
metadata:
creationTimestamp: null
name: sandbox
labels:
environment: sandbox
Question 2: Write a Kubernetes YAML manifest to create a new namespace named “dev” and set a resource quota that limits the total CPU usage to 4 cores and the total memory usage to 2 GB.
apiVersion: v1
kind: Namespace
metadata:
name: dev
---
apiVersion: v1
kind: ResourceQuota
metadata:
name: dev-quota
namespace: dev
spec:
hard:
cpu: "4"
memory: 2Gi
Question 3: Write a Kubernetes YAML manifest to create a new namespace named “production” and create a service account named “prod-service-account” within that namespace.
apiVersion: v1
kind: Namespace
metadata:
name: production
---
apiVersion: v1
kind: ServiceAccount
metadata:
name: prod-service-account
namespace: production
Question 4: Write a YAML manifest file to create a Kubernetes Pod with a single container running an Nginx web server. Set the container image to the latest version of Nginx and expose port 80 for incoming traffic.
apiVersion: v1
kind: Pod
metadata:
creationTimestamp: null
labels:
run: nginx
name: nginx
spec:
containers:
- image: nginx
name: nginx
ports:
- containerPort: 80
resources: {}
dnsPolicy: ClusterFirst
restartPolicy: Always
Question 5: Write a YAML manifest file to create a Kubernetes Pod with two containers: one running a Python application and the other running a Redis database. Define the necessary environment variables and volume mounts for the containers to interact with each other. Solution
Question 6: Write a YAML manifest file to create a Kubernetes Pod with resource requests and limits set for CPU and memory. Specify the container image, command, and arguments to run a custom application within the Pod.
apiVersion: v1
kind: Pod
metadata:
labels:
run: test-pod
name: test-pod
namespace: sandbox
spec:
containers:
- command: ["sh", "-c", "sleep 100"]
image: busybox
name: test-pod
resources:
limits:
cpu: 2
memory: 200Mi
requests:
cpu: 1
memory: 100Mi
dnsPolicy: ClusterFirst
Question 7: Write a YAML manifest file to create a Kubernetes Pod with an init container that creates a file foo in /foo location before the main container starts. Ensure that the init container completes successfully before the main container initializes. In the main container print the contents of /foo file.
Question 8: Write a YAML manifest file to create a Kubernetes Pod that pulls images from a private container registry. Configure the necessary authentication credentials and secret references to allow the Pod to authenticate and pull the private images.
Solution:

To run a pod, which uses an image from the private repository, you need to do
two things:
Create a Secret holding the credentials for the Docker registry.
- Reference that Secret in the imagePullSecrets field of the pod manifest.
kubectl create secret docker-registry mydockersecret \
--docker-username=username --docker-password=password \
--docker-email=email@provider.com
Reference that Secret in the imagePullSecrets field of the pod manifest.
apiVersion: v1
kind: Pod
metadata:
name: private-pod
spec:
imagePullSecrets:
- name: mydockersecret
containers:
- image: username/imagename:tag
name: container-name

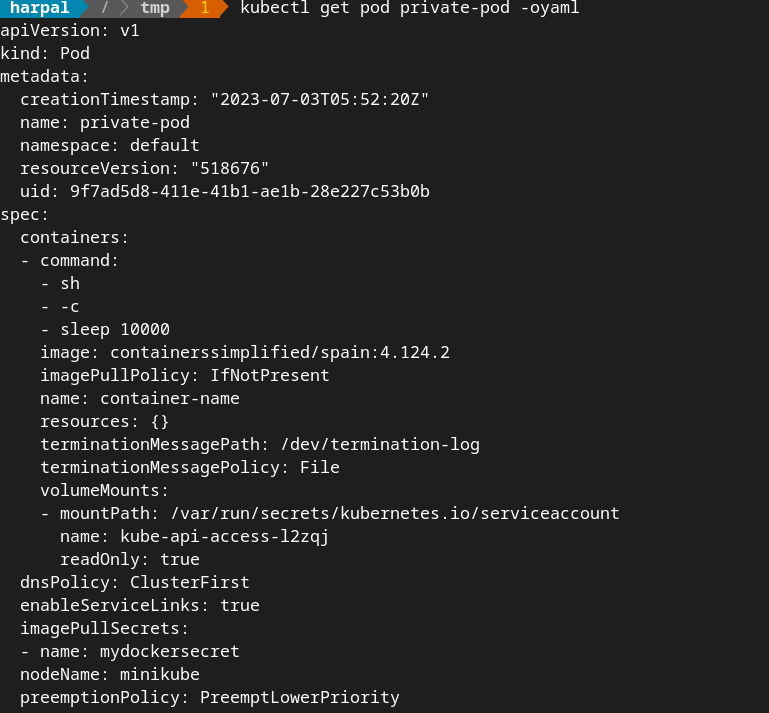
Question 9: Write a Kubernetes YAML manifest to create a new service account named “test-account” in the default namespace.
apiVersion: v1
kind: ServiceAccount
metadata:
creationTimestamp: null
name: test-account
Question 10: Write a Kubernetes YAML manifest to create a new service account named “test-account” in a specific namespace named “test-namespace”.
apiVersion: v1
kind: ServiceAccount
metadata:
creationTimestamp: null
name: test-account
namespace: test-namespace
Question 11: Write a Kubernetes YAML manifest to create a new service account named “backend-service-account” and assign it the “system:serviceaccount:default:my-service-account” role.
apiVersion: v1
kind: ServiceAccount
metadata:
creationTimestamp: null
name: backend-service-account
---
apiVersion: rbac.authorization.k8s.io/v1
kind: RoleBinding
metadata:
creationTimestamp: null
name: test-role
roleRef:
apiGroup: rbac.authorization.k8s.io
kind: Role
name: system:serviceaccount:default:my-service-account
subjects:
- kind: ServiceAccount
name: backend-service-account
namespace: default
Question 12: Write a Kubernetes YAML manifest to create a new service account named “worker-service-account” and bind it to the “cluster-admin” role, granting full administrative access to the cluster.
apiVersion: v1
kind: ServiceAccount
metadata:
name: worker-service-account
apiVersion: rbac.authorization.k8s.io/v1
kind: ClusterRoleBinding
metadata:
name: test-role
roleRef:
apiGroup: rbac.authorization.k8s.io
kind: ClusterRole
name: cluster-admin
subjects:
- kind: ServiceAccount
name: worker-service-account
namespace: default
Question 13: Write a Kubernetes YAML manifest to create a new service account named “deploy-service-account” and give it read-only access to the secrets in the “kube-system” namespace.
Question 14: Write a Kubernetes YAML manifest to create a Deployment resource named “nginx-deployment” with five replicas, using the “nginx” container image.
Question 15: Write a Kubernetes YAML manifest to create a Service resource named “my-service” that exposes port 9090 and targets the pods labeled with “app=my-app”.
Question 16: Write a Kubernetes YAML manifest to create a PersistentVolume resource named “my-pv” with a capacity of 5GB and using the “hostPath” volume type.
Question 17: Write a Kubernetes YAML manifest to create a PersistentVolumeClaim resource named “my-pvc” with a request for 2GB storage.
Question 18: Write a Kubernetes YAML manifest to create a ConfigMap resource named “my-config” with key-value pairs for configuration data.
Question 19: Write a Kubernetes YAML manifest to create a Deployment named “my-deployment” with a container that has a CPU request of 0.75 cores and a memory request of 256MB.
Question 20: Write a Kubernetes YAML manifest to create a Pod named “my-pod” with a container that has a CPU limit of 2 cores and a memory limit of 2GB.
Question 21: Write a Kubernetes YAML manifest to create a Deployment named “my-deployment” with two containers: “frontend” with a CPU request of 0.4 cores and a memory request of 256MB, and “backend” with a CPU request of 0.75 cores and a memory request of 512MB.
Question 22: Write a Kubernetes YAML manifest to create a Pod named “my-pod” with a container that has a CPU limit of 0.5 cores and a memory limit of 512MB, and also specify a CPU request of 0.2 cores and a memory request of 256MB.
Question 23: Write a Kubernetes YAML manifest to create a Deployment named “my-deployment” with a container that has a CPU limit of 4 cores and a memory limit of 4GB, and also specify a CPU request of 2 cores and a memory request of 2GB.
Question 24: Write a Kubernetes YAML manifest to create a network policy that allows incoming traffic to a Pod labeled “app=my-app” on port 80 from any source.
Question 25: Write a Kubernetes YAML manifest to create a network policy that allows incoming traffic to Pods labeled “tier=frontend” on ports 8080 and 8443 only from Pods in the same namespace.
Question 26: Write a Kubernetes YAML manifest to create a network policy that denies incoming traffic to a specific Pod labeled “access=blocked” from any source.
Question 27: Write a Kubernetes YAML manifest to create a network policy that allows incoming traffic to Pods labeled “tier=backend” on port 8080 from Pods in a different namespace named “sandbox”.
Question 28: Write a Kubernetes YAML manifest to create a network policy that allows incoming traffic to a specific Pod labeled “app=my-app” from a specific IP range, such as 192.168.0.0/24.
Question 29: Write a PodSecurityPolicy that restricts the ability of Pods to run privileged containers.
Question 30: Write a PodSecurityPolicy that restricts the ability of Pods to access the host filesystem.
Question 31: Write a PodSecurityPolicy that restricts the ability of Pods to run containers with the NET_RAW
capability.
Question 32: Write a PodSecurityPolicy that restricts the ability of Pods to run containers with the SYS_ADMIN
capability.
Question 33: Write a PodSecurityPolicy that restricts the ability of Pods to mount host volumes.
Question 34: Write a Kubernetes YAML manifest to create a Job named “my-job” that runs a container with the image “busybox” and command “sleep 1000”.
Question 35: Write a Kubernetes YAML manifest to create a Job named “my-backup-job” that runs a container with the image “my-backup-container” and mounts a PersistentVolumeClaim named “my-data-pvc” as a volume.
Question 36: Write a Kubernetes YAML manifest to create a Job named “batch-processing” that runs multiple parallel pods with the image “my-batch-processor” and processes data from a ConfigMap named “my-batch-config”.
Question 37: Write a Kubernetes YAML manifest to create a Job named “cleanup-job” that runs a container with the image “cleanup-container” and performs cleanup tasks on specific resources.
Question 38: Write a Kubernetes YAML manifest to create a CronJob named “my-backup-cron” that schedules a backup Job to run every day at 5 AM.
Question 39: Write a Kubernetes YAML manifest to create a Deployment named “my-deployment” with a container that sets the environment variable “MYSQL_DATABASE_URL” to “mysql://user:password@hostname:port/database”.
Question 40: Write a Kubernetes YAML manifest to create a Pod named “my-pod” with multiple containers. Set the environment variable “MY_API_KEY” to “12345” in one container and “MY_DB_PASSWORD” to “secretpassword” in another container.
Question 41: Write a Kubernetes YAML manifest to create a Deployment named “my-deployment” that reads environment variables from a ConfigMap named “my-config-map” and sets them in the container.
Question 42: Write a Kubernetes YAML manifest to create a Pod named “my-pod” with a container that uses an environment variable from a Secret named “my-secret”. Set the variable “API_KEY” to the value stored in the Secret.
Question 43: Write a Kubernetes YAML manifest to create a Deployment named “my-deployment” that uses downward API to expose the pod’s own IP address as an environment variable named “POD_IP”.
Question 44: Write a Kubernetes YAML manifest to create a ConfigMap named “my-config-map” with key-value pairs for database connection details such as “DB_HOST”, “DB_PORT”, “DB_USER”, and “DB_PASSWORD”.
Question 45: Write a Kubernetes YAML manifest to create a Deployment named “my-deployment” that mounts the ConfigMap “my-config-map” as environment variables in a container.
Question 46: Write a Kubernetes YAML manifest to create a ConfigMap named “my-app-config” by reading data from a file named “app.properties” located in the same directory.
Question 47: Write a Kubernetes YAML manifest to create a ConfigMap named “app-config” that contains configuration data as key-value pairs and use it to set the command-line arguments for a container in a Pod.
Question 48: Write a Kubernetes YAML manifest to create a ConfigMap named “my-configmap” with key-value pairs and mount it as a volume in a Pod, allowing an application to read the configuration data from the mounted file.
Question 49: Write a Kubernetes YAML manifest to create a Secret named “my-secret” with a single key-value pair for a database password.
Question 50: Write a Kubernetes YAML manifest to create a Deployment named “my-deployment” that mounts the Secret “my-secret” as environment variables in a container.
Question 51: Write a Kubernetes YAML manifest to create a Secret named “ssl-certificate” that reads the certificate data from a file named “cert.pem” and the corresponding private key from a file named “key.pem”.
Question 52: Write a Kubernetes YAML manifest to create a Secret named “db-credentials” with separate keys for the database username and password.
Question 53: Write a Kubernetes YAML manifest to create a Secret named “api-keys” with multiple keys for different API keys used by an application.
Question 54: Write a Kubernetes YAML manifest to create a Pod named “my-pod” that mounts an emptyDir volume named “my-volume” at the path “/data” within the container.
Question 55: Write a Kubernetes YAML manifest to create a Deployment named “my-deployment” that mounts a PersistentVolumeClaim named “my-pvc” as a volume in the container.
Question 56: Write a Kubernetes YAML manifest to create a Pod named “my-pod” that mounts a ConfigMap named “my-config” as a volume and reads the configuration data from the mounted volume.
Question 57: Write a Kubernetes YAML manifest to create a Deployment named “my-deployment” that mounts a Secret named “my-secret” as a volume and uses the secret data within the container.
Question 58: Write a Kubernetes YAML manifest to create a Pod named “my-pod” that mounts a hostPath volume at the path “/host-data” on the node and makes the host’s file system content accessible within the container.
Question 59: Write a Kubernetes YAML manifest to create a Service named “my-service” that exposes port 9090 and targets pods labeled with “app=my-app”.
Question 60: Write a Kubernetes YAML manifest to create a Service named “frontend-service” that exposes ports 8080 and 8443 and uses a LoadBalancer type to distribute traffic to the frontend pods.
Question 61: Write a Kubernetes YAML manifest to create a Service named “my-database-service” that exposes port 3306 and targets a specific pod IP address for a database backend.
Question 62: Write a Kubernetes YAML manifest to create a Service named “my-internal-service” that is only accessible within the cluster, using the ClusterIP type.
Question 63: Write a Kubernetes YAML manifest to create a Service named “my-headless-service” that does not assign a cluster IP and instead returns the IP addresses of the pods directly when queried.
Question 64: Write a Kubernetes YAML manifest to create an Ingress resource named “my-ingress” that routes traffic to a Service named “my-service” on port 80.
Question 65: Write a Kubernetes YAML manifest to create an Ingress resource named “my-api-ingress” that routes traffic to different backend Services based on the URL path. Requests to “/api/v1” should be forwarded to “my-api-service” on port 8080, and requests to “/api/v2” should be forwarded to “my-api-service-v2” on port 8080.
Question 66: Write a Kubernetes YAML manifest to create an Ingress resource named “secure-ingress” that enables HTTPS termination and routes traffic to a Service named “my-secure-service” on port 8443. Use a TLS secret named “my-tls-secret” to secure the connection.
Question 67: Write a Kubernetes YAML manifest to create an Ingress resource named “my-subdomain-ingress” that routes traffic to different backend Services based on subdomains. Requests to “myapp1.example.com” should be forwarded to “myapp1-service” on port 8080, and requests to “myapp2.example.com” should be forwarded to “myapp2-service” on port 8080.
Question 68: Write a Kubernetes YAML manifest to create an Ingress resource named “my-rewrite-ingress” that rewrites the URL path before forwarding traffic to a backend Service. Requests to “/blog” should be rewritten to “/posts” and forwarded to “my-blog-service” on port 80.
Question 69: Write a Kubernetes YAML manifest to create a Deployment named “my-deployment” that runs a single container with the image “my-app” and sets the replicas to four.
Question 70: Write a Kubernetes YAML manifest to create a Deployment named “my-frontend-deployment” that runs multiple containers with the images “nginx:1.25-alpine3.17-slim” and “nginx:1.25-alpine” and performs a rolling update.
Question 71: Write a Kubernetes YAML manifest to create a Deployment named “my-deployment” that sets resource limits for CPU and memory usage in the container.
Question 72: Write a Kubernetes YAML manifest to create a Deployment named “my-deployment” that mounts a ConfigMap named “my-config” as environment variables in the container.
Question 73: Write a Kubernetes YAML manifest to create a Deployment named “my-deployment” that uses a Secret named “my-secret” as a volume and mounts it at a specific path within the container.
Question 74: Write a Kubernetes YAML manifest to create a Deployment named “my-deployment” and configure Horizontal Pod Autoscaling based on CPU utilization, targeting an average utilization of 80%.
Question 75: Write a Kubernetes YAML manifest to create a Deployment named “backend-deployment” and configure Horizontal Pod Autoscaling based on custom metrics, such as the number of requests per second, with a target value of 100 requests per second.
Question 76: Write a Kubernetes YAML manifest to create a HorizontalPodAutoscaler resource named “my-hpa” that scales a Deployment named “my-deployment” between 2 and 10 replicas based on memory utilization, targeting an average utilization of 75%.
Question 77: Write a Kubernetes YAML manifest to create a Deployment named “my-frontend-deployment” and configure Horizontal Pod Autoscaling based on both CPU and memory utilization, with different target utilization values for each metric.
Question 78: Write a Kubernetes YAML manifest to create a HorizontalPodAutoscaler resource named “my-hpa” that scales a Deployment named “my-deployment” based on a custom external metric, such as the number of items in a message queue, with a target value of 500 items.
Question 79: Write a Kubernetes YAML manifest to create a headless Service named “my-service” that selects pods with the label “app=my-app” and does not assign a cluster IP.
Question 80: Write a Kubernetes YAML manifest to create a headless Service named “my-database-service” that selects pods with the label “tier=database” and sets the selector to match a specific label.
Question 81: Write a Kubernetes YAML manifest to create a headless Service named “backend-service” that selects pods with the label “app=backend” and forwards traffic to a specific port on the pods.
Question 82: Write a Kubernetes YAML manifest to create a headless Service named “my-cache-service” that selects pods with the label “tier=cache” and specifies a custom DNS domain name for the service.
Question 83: Write a Kubernetes YAML manifest to create a headless Service named “my-custom-service” that selects pods based on a specific label selector and sets a specific timeout value for DNS resolution.
Question 84: Write a Kubernetes YAML manifest to create a Deployment named “my-deployment” with a readiness probe that performs an HTTP GET request to the “/health” endpoint every 8 seconds.
Question 85: Write a Kubernetes YAML manifest to create a Deployment named “my-backend-deployment” with a liveness probe that executes a command to check if a specific process is running inside the container every 12 seconds.
Question 86: Write a Kubernetes YAML manifest to create a Deployment named “my-deployment” with both a readiness probe and a liveness probe. The readiness probe should perform a TCP socket check on port 8080, and the liveness probe should execute an HTTP GET request to the “/healthz” endpoint.
Question 87: Write a Kubernetes YAML manifest to create a Deployment named “my-deployment” with a readiness probe that waits for a specific file to exist in a shared volume mounted in the container.
Question 88: Write a Kubernetes YAML manifest to create a Deployment named “my-deployment” with a liveness probe that performs an HTTP GET request to the “/ping” endpoint every 12 seconds, with a failure threshold of 3 before considering the container unhealthy.
Question 89: Write a Kubernetes YAML manifest to create a StatefulSet named “my-stateful-set” that runs a single replica of a pod with a specified image and labels.
Question 90: Write a Kubernetes YAML manifest to create a StatefulSet named “my-db-stateful-set” with three replicas, each with a specific pod template, and persistent storage for data.
Question 91: Write a Kubernetes YAML manifest to create a StatefulSet named “my-web-stateful-set” that uses a headless service for domain name resolution and has an ordered pod termination policy.
Question 92: Write a Kubernetes YAML manifest to update an existing StatefulSet named “my-stateful-set” by increasing the number of replicas from 2 to 4.
Question 93: Write a Kubernetes YAML manifest to create a StatefulSet named “cache-stateful-set” that uses a ConfigMap to customize the configuration of the pods.
Question 94: Write a Kubernetes YAML manifest to create a Deployment named “my-deployment” that runs a container with the image “my-app” and sets a command-line argument of “–env=prod”.
Question 95: Write a Kubernetes YAML manifest to create a Deployment named “backend-deployment” that runs a container with the image “backend-app” and sets multiple command-line arguments, such as “–port=8080” and “–debug=true”.
Question 96: Write a Kubernetes YAML manifest to create a Deployment named “worker-deployment” that runs a container with the image “worker-app” and sets an argument to pass a specific input file path, such as “–input=/data/input.txt”.
Question 97: Write a Kubernetes YAML manifest to create a Deployment named “frontend-deployment” that runs a container with the image “frontend-app” and sets an argument to specify a custom log level, such as “–log-level=debug”.
Question 98: Write a Kubernetes YAML manifest to create a Deployment named “api-deployment” that runs a container with the image “api-app” and sets an argument to provide a database connection string, such as “–db connection=postgres://user:password@hostname:port/database”.
Question 99: Write a Kubernetes YAML manifest to create a Pod named “my-pod” with a security context that sets the runAsUser to a specific user ID, such as 1001.
Question 100: Write a Kubernetes YAML manifest to create a Deployment named “web-deployment” with a security context that enables the readOnlyRootFilesystem setting for the containers.
Question 101: Write a Kubernetes YAML manifest to create a Pod named “privileged” with a security context that sets the privileged option to true, granting elevated privileges to the containers.
Question 102: Write a Kubernetes YAML manifest to create a Pod named “restricted-pod” with a security context that sets the seccompProfile to a specific profile name, enabling additional kernel-level security restrictions.
Question 103: Write a Kubernetes YAML manifest to create a Deployment named “controlled-deployment” with a security context that enables the runAsNonRoot setting for the containers, ensuring they run as non-root users.
Question 104: Write a Kubernetes YAML manifest to create a Pod named “test-pod” with a privileged container that has unrestricted access to the host system.
Question 105: Write a Kubernetes YAML manifest to create a Deployment named “backend-deployment” with a privileged container that requires host network access for low-level network operations.
Question 106: Write a Kubernetes YAML manifest to create a Pod named “privileged-pod” with a privileged container that needs access to specific devices on the host, such as /dev/sda
. Try to list files of /dev directory from inside the container.
Question 107: Write a Kubernetes YAML manifest to create a Deployment named “privileged-test-deployment” with multiple containers, where one container is privileged and the others are not, ensuring isolation of privileges.
Question 108: Write a Kubernetes YAML manifest to create a Pod named “privileged-test-pod” with a privileged container that requires the ability to mount and modify host directories, such as /var/log
for log file access.
Question 109: Write a Kubernetes YAML manifest to create a Service that exposes a Pod’s port, but due to misconfiguration, causes a loopback error when attempting to access the Service from within the same cluster.
Question 110: Write a Kubernetes YAML manifest to create a Pod with multiple containers, where one container makes a request to another container within the same Pod, but due to misconfiguration, results in a loopback error.
Question 111: Write a Kubernetes YAML manifest to create an Ingress resource that routes traffic to multiple Services within the same cluster, but due to misconfiguration, triggers loopback errors when accessing the Services through the Ingress.
Question 112: Write a Kubernetes YAML manifest to create a Deployment with a readiness probe that makes a request to the same Pod, causing a loopback error due to misconfiguration.
Question 113: Write a Kubernetes YAML manifest to create a Pod with a sidecar container that communicates with the main container using localhost, but due to misconfiguration, leads to loopback errors.
Question 114: Write a Kubernetes YAML manifest to create a Pod that mounts a secret named “my-test-secret” as a volume and mounts it at the path “/app/secrets” within the container.
Question 115: Write a Kubernetes YAML manifest to create a Deployment that mounts a secret named “my-database-secret” as a volume and uses it to provide the database credentials to the container.
Question 116: Write a Kubernetes YAML manifest to create a StatefulSet that mounts a secret named “my-tls-secret” as a volume and uses it to provide TLS certificates to the container.
Question 117: Write a Kubernetes YAML manifest to create a Pod that mounts multiple secrets as volumes, such as “my-db-secret” and “my-api-secret”, and uses them within the container for different purposes.
Question 118: Write a Kubernetes YAML manifest to create a Deployment that mounts a secret named “my-config-secret” as a volume and uses it to provide configuration files to the container.
Question 119: Write a Kubernetes YAML manifest to create a ServiceAccount named “my-service-account” and generate a token for authentication purposes.
Question 120: Write a Kubernetes YAML manifest to create a Pod that uses a specific ServiceAccount named “my-backend-service-account” and mounts the associated token as a volume.
Question 121: Write a Kubernetes YAML manifest to create a RoleBinding that grants a ServiceAccount named “my-frontend-sa” access to a specific role or cluster role.
Question 122: Write a Kubernetes YAML manifest to create a Pod with an init container that retrieves a token from an external source and passes it to the main container for authentication.
Question 123: Write a Kubernetes YAML manifest to create a Secret that stores a token and use it as a bearer token for authenticating requests to an external API within a Pod.
Question 124: Write a Kubernetes YAML manifest to create a new namespace named “staging” and configure network policies to only allow incoming traffic from the “frontend” namespace on port 80.
Question 125: Write a YAML manifest file to create a ClusterRole in Kubernetes that allows a user to create, update, and delete ConfigMaps and Secrets across all namespaces in the cluster. Test the ClusterRole by creating and modifying ConfigMaps and Secrets.
Question 126: Write a YAML manifest file to create a ClusterRole in Kubernetes that allows listing and accessing all Pods and Services within a specific namespace. Test the ClusterRole by querying and interacting with Pods and Services in the designated namespace.
Question 127: Write a YAML manifest file to create a Kubernetes Node with a taint that repels all Pods by default. Deploy a Pod and observe how it gets scheduled or affected by the Node’s taint.
Question 128: Write a YAML manifest file to create a Kubernetes Node with a taint that allows only Pods with specific toleration to be scheduled. Deploy Pods with and without the corresponding toleration and observe their scheduling behavior.
Question 129: Write a YAML manifest file to add a taint to an existing Kubernetes Node. Update the tolerations of existing Pods to match the taint and ensure they continue to run on the tainted Node.
Question 130: Write a YAML manifest file to create a Kubernetes Deployment that specifies toleration for a specific taint. Ensure that the Pods created by the Deployment are scheduled only on Nodes that have the corresponding taint.
Question 131: Write a YAML manifest file to create a Kubernetes Node with multiple taints. Deploy Pods with different tolerations and observe how they get scheduled or affected by the various taints.
Question 132: Write a YAML manifest file to create a PersistentVolumeClaim in Kubernetes with a specific storage class and storage size. Create a Pod that mounts the PVC as a volume and write a simple file to the mounted directory.
Question 133: Write a YAML manifest file to create a PVC in Kubernetes with access mode “ReadWriteMany” and a storage size of 5GB. Create multiple Pods that mount the PVC as a volume simultaneously and verify that they can read and write data concurrently.
Question 134: Write a YAML manifest file to create a PVC in Kubernetes and bind it to an existing PersistentVolume that has specific access modes and storage capacity. Create a Pod that uses the PVC as a volume and verify that it can access the data stored in the PersistentVolume.
Question 135: Write a YAML manifest file to create a PVC in Kubernetes with a specific storage class and access mode. Update the PVC’s storage size and verify that the change is reflected in the Pod that uses the PVC as a volume.
Question 136: Write a YAML manifest file to create a PVC in Kubernetes that requests storage from a dynamically provisioned PersistentVolume. Create a Pod that mounts the PVC as a volume and write a script to continuously monitor the available storage space in the PVC.
Question 137: Use the kubectl
command to list all Pods running in a specific namespace in the cluster.
Question 138: Use the kubectl
command to create a Deployment with three replicas, using a YAML manifest file.
Question 139: Use the kubectl
command to scale up a Deployment to five replicas.
Question 140: Use the kubectl
command to describe a Service and inspect its details, such as the associated Pods and endpoints.
Question 141: Use the kubectl
command to apply changes to an existing Deployment, such as updating the container image tag or modifying environment variables.
Question 142: Use the kubectl
command to create a new namespace “sandbox” in the cluster.
Question 143: Use the kubectl
command to execute the command “top” inside a running container based on the “Ubuntu” image in a Pod.
Question 144: Use the kubectl
command to label a group of Nodes in the cluster with a custom label “dev”.
Question 145: Write a YAML manifest file to create a Deployment in Kubernetes with a custom annotation that provides additional metadata about the Deployment. Use the kubectl
command to retrieve and display the annotations for the Deployment.
Question 146: Write a YAML manifest file to create a DaemonSet in Kubernetes that deploys a specific container image to all nodes in the cluster. Use the kubectl
command to verify that the DaemonSet pods are running on each node.
Question 147: Use the kubectl
command to list all Pods in a specific namespace that has a certain label key-value pair.
Question 148: Write a kubectl
command to scale up a Deployment in Kubernetes, targeting only the Pods that have a specific label.
Question 149: Write a kubectl
command to label all Pods in a specific namespace with a custom label, without affecting any other labels already applied to the Pods.
Question 150: Write a YAML manifest file to create a Pod in Kubernetes with a specific nodeSelector
that ensures the Pod is scheduled only on nodes with a specific label. Use the kubectl
command to verify that the Pod is running on the appropriate node.